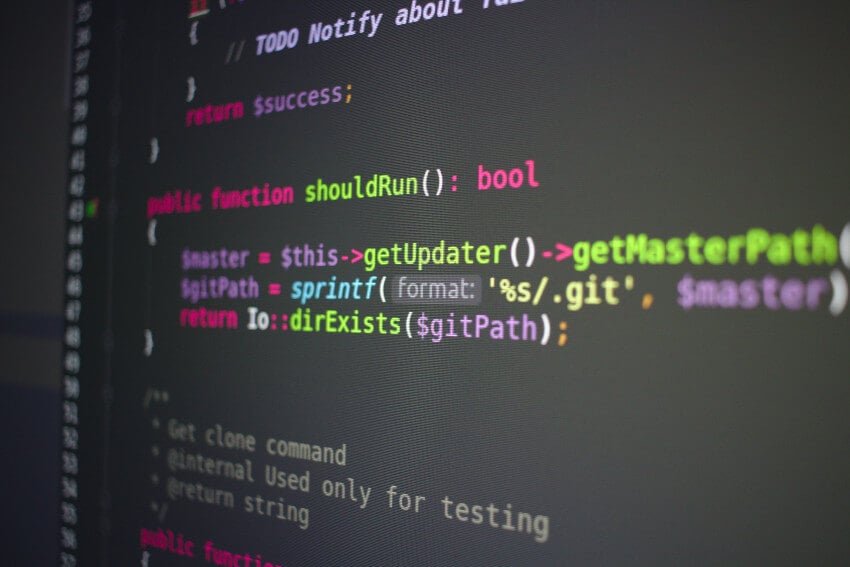
PHP 8.2: What’s New and Improved
PHP is a popular programming language used for web development, and its latest version. The PHP 8.2, brings a host of new features and improvements. In this post, we’ll take a look at what’s new in PHP 8.2 and how it compares to the previous version, PHP 7.4, with code examples.
PHP 8.2 “match” expression:
One of the biggest new features in PHP 8.2 is the addition of the “match” expression. This new syntax allows developers to easily match a value against a set of patterns. Similar to how a “switch” statement works. The “match” expression is more concise and easier to read than a series of “if” statements. Making it a valuable addition for developers. Here is an example of how to use the “match” expression:
$value = 5;
// Traditional if-elseif-else statement
if ($value === 1) {
$result = 'one';
} elseif ($value === 2) {
$result = 'two';
} else {
$result = 'other';
}
// Same thing using match expression
$result = match ($value) {
1 => 'one',
2 => 'two',
default => 'other',
};
PHP 8.2 “__debugInfo” magic method:
Another notable feature in PHP 8.2 is the addition of the “__debugInfo” magic method. This method allows developers to customize the output of the “var_dump” and “print_r” functions when debugging their code. This can be particularly useful when working with large or complex objects, as it allows developers to see only the most relevant information. Here is an example of how to use the “__debugInfo” magic method:
class User
{
private $name;
private $age;
private $email;
public function __construct(string $name, int $age, string $email)
{
$this->name = $name;
$this->age = $age;
$this->email = $email;
}
public function __debugInfo()
{
return [
'name' => $this->name,
'email' => $this->email,
];
}
}
$user = new User('John Doe', 30, '[email protected]');
var_dump($user);
// Outputs:
// object(User)#1 (2) {
// ["name"]=>
// string(8) "John Doe"
// ["email"]=>
// string(18) "[email protected]"
// }
PHP 8.2 also includes a number of deprecations and bug fixes. Some notable deprecations include the removal of the “create_function” function and the “__autoload” magic method. These features have been replaced by more modern alternatives, such as anonymous functions and the “spl_autoload_register” function.
In terms of bug fixes, PHP 8.2 includes fixes for a number of issues related to type coercion, exception handling, and the core language itself. These fixes should help improve the stability and performance of PHP applications.
PHP 8.2 Deprecations: What’s Being Removed in the Latest Version
One notable deprecation in PHP 8.2 is the removal of the “create_function” function. This function allowed developers to create anonymous functions on the fly, but it has been removed in favor of more modern alternatives such as anonymous functions. Here is an example of how to use anonymous functions in PHP 8.2:
// Old way using create_function
$plusTwo = create_function('$x', 'return $x + 2;');
// New way using anonymous functions
$plusTwo = fn($x) => $x + 2;
Another deprecation in PHP 8.2 is the removal of the “__autoload” magic method. This method allowed developers to automatically load PHP classes when they were needed, but it has been replaced by the more modern “spl_autoload_register” function. Here is an example of how to use “spl_autoload_register” in PHP 8.2:
// Old way using __autoload
function __autoload($className)
{
require_once $className . '.php';
}
// New way using spl_autoload_register
spl_autoload_register(function($className) {
require_once $className . '.php';
});
PHP 8.2 also includes a number of other deprecations, such as the removal of the “each” function and the “PHP_INT_SIZE” and “PHP_INT_MIN” predefined constants. Developers should take note of these deprecations and update their code accordingly.
The deprecations in PHP 8.2 are a necessary step in the evolution of the language. While it may require some work to update your code, the removal of outdated or less-used features helps to keep PHP modern and efficient. So, it is always a good idea to keep an eye on the deprecation list when upgrading to a new version of PHP.
Overall, PHP 8.2 is a solid update that brings a number of useful new features and improvements. Whether you’re a seasoned PHP developer or just starting out, it’s worth taking a look at what PHP 8.2 has to offer. So, upgrade to the new version and take advantage of all these new features and improvements.